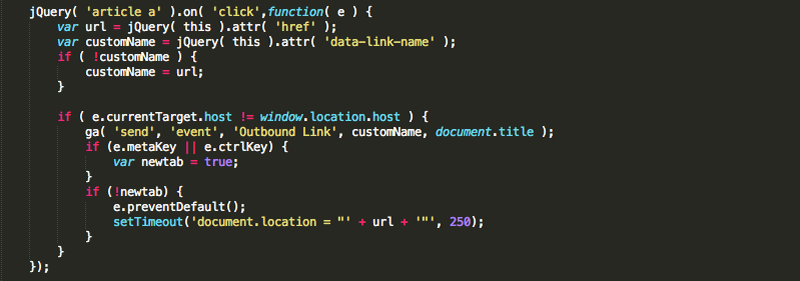
I was recently generating some new server and email passwords, and realized that while most of the strong password generators available online work great for desktops, they aren’t very user friendly for entering on iOS or mobile devices. Often you can find a few checkboxes and sliders for those tools to make it a bit more iPhone friendly, but they don’t always work. Ideally a password that you frequently use on an iPhone or other iOS device should be “strong” (i.e. have possibly a symbol or two and a mix of upper and lower case characters), but should also be fairly easy to type.
So this morning I decided to write a bit of PHP to generate an iOS friendly strong password. Basically the idea is to only use mostly keys that are accessible without having to hit shift or the keyboard button that switches to symbol mode.
function generate_password( $length = 10 ) { // Enforce a minimum length of 10 symbols $length = max( 10, $length ); $password = ''; $available_symbols = array(); // These only require one tap per symbol $available_symbols[] = 'qwertyuiopasdfghjklzxcvbnm'; // These require two taps per symbol $available_symbols[] = 'QWERTYUIPASDFGHJKLZXCVBNM1234567890!$?'; // These require three taps per symbol $available_symbols[] = '[]{}#%^*+=_/|~<>?!'; // Remove this next line if you only want mostly easy-to-type passwords $password .= $available_symbols[2][ mt_rand( 0, strlen( $available_symbols[2] ) - 1 ) ]; // These two require two keypresses each, but make the password much stronger $password .= $available_symbols[1][ mt_rand( 0, strlen( $available_symbols[1] ) - 1 ) ]; $password .= $available_symbols[1][ mt_rand( 0, strlen( $available_symbols[1] ) - 1 ) ]; for ( $i = 0; $i < $length - strlen( $password ); $i++ ) { $password .= $available_symbols[0][ mt_rand( 0, strlen( $available_symbols[0] ) ) - 1 ]; } return str_shuffle( $password ); }
Basically I’ve included only one symbol that uses three taps to produce, two symbols that use two taps to produce, with the remainder being lowercase symbols that only take one tap to produce. That way these passwords are still essentially strong, but also minimize the number of taps it takes to reproduce them on mobile devices.
I removed a few symbols that were a bit ambiguous, and removed a few others that just impeded the readability of the password. I’m sure this code can be simplified, but I purposefully made it easy to read as opposed to really efficient.
This page is purposefully not cached, so if you are looking for an iPhone or iPad friendly strong password, you can use one of these:
- Password 10 Symbols Long: [strongpw length=”10″]
- Password 12 Symbols Long: [strongpw length=”12″]
- Password 14 Symbols Long: [strongpw length=”14″]
If you find this useful, let me know.
Interesting idea. Do you think that those passwords will be more predictable and maybe less strong?
I’m biased but I think StrongPasswordGenerator.com is a better bet for creating passwords, for any device. It’s responsively designed so it should be usable on any device.
I think they are marginally less strong, but the trade off of course is that they are easier to type. So I think for iPhone or iOS users it’s a trade-off many would be happy to make.
I just checked on HowSecureIsMyPassword using these. It said it would take 14 years to crack the 10 character one on a desktop PC, 64,000 years for the 12 character one, and 290 million years for the 14 character one. I think that’s good enough for most people.
The DropBox guys recently released a library to estimate how good passwords actually are as well, and WordPress recently integrated their library. You can read about it here – Realistic Password Strength Estimation.
You can test out the result of that library here. The 10, 12, and 14 character passwords generated above (in my test) all scored 4/4 and would take “centuries” to crack.